Dynamically Generating Document Samples with Acrobat Services | by Raymond Camden
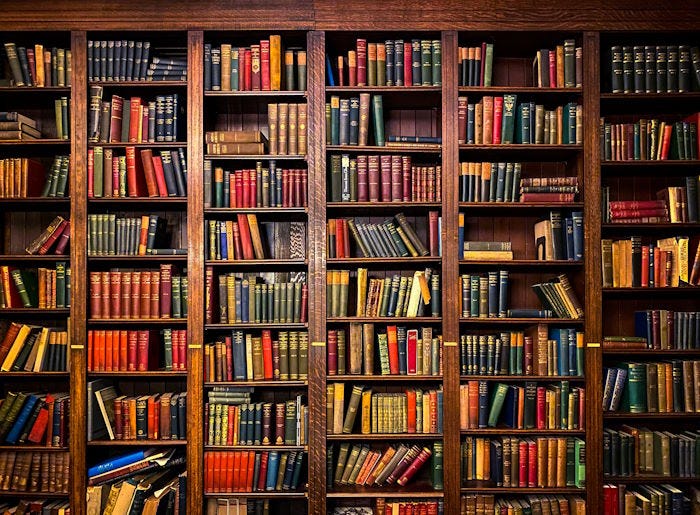
Many organizations offer documents for sale, and as part of that process, they will sometimes create a sample of the document to offer online for free.
As an example, an online technical bookseller may offer the first thirty pages as a ‘teaser’ to help encourage sales.
Creating these samples could be done by hand, but this is a great example of where Adobe Acrobat Services can help automate the process.
In this blog, we’ll demonstrate a simple example of this process. We’ll use Node.js, but the principals shown here could be done in any language via the REST APIs. Let’s get started!
Before going into the code, let’s quickly cover what exactly is being built.
For the purpose of this particular demo, the process of creating samples will be done via a Node.js script that reads from one folder and saves it to another.
This is, obviously, not automated, but could be migrated to a platform where that would be allowed.
For example, this same process could be done in Microsoft PowerAutomate and set up to automatically kick off when a new file is added.
Our process will do two things to each PDF:
Our code is split between two files.
The first file is responsible for checking the directories (both input and output) to figure out what it needs to do. Once it determines the PDFs that need to be actually processed, it calls that routine which is set up in the second file.
Here’s the code for that first file:
From the top, you can see our configuration variables pointing to the input and output directories, as well as the PDF that’s placed in front of each sample result.
Also, make note of the SAMPLE_SIZE
variable which determines the number of pages to use when creating the sample. It would be possible to make this dynamic.
So for example, the PDF Properties API could be used to figure out the total number of pages, and math could then be used to generate a relative size for the number of pages. Perhaps 10% or so, with an upward maximum bound of some limit. For this example, we’ll keep it to a simple 5-page max.
To run quicker, the script checks to see if a corresponding file of the same name from the input directory already exists in the output and skips over it. Finally, when it figures out what needs to be done, it iterates over each and calls makeSample
in our next script. Let’s now take a look at that.
The script, makeSamples.js
, is essentially a wrapper for our REST APIs. In general, the process of working with the Acrobat Services APIs all follow the same basic flow:
This may sound a bit complex, but each part is a relatively small REST call that’s easy to write. Here’s the makeSample
function:
Let’s break this down. First, getting an access token:
This is code we’ve shared before. Essentially the client ID and secret and exchanged for the token. Do note we added a bit of caching (cachedToken
) so that we can reuse the value.
Next, the upload
function, which is actually two bits – the creation of an “Asset” and the actual upload itself. This function simplifies it into one:
Which then chains to the two utility functions:
So far, everything has basically been “setup” and preparation for the real work: actually creating the sample.
Luckily we can do this in one quick call to the Combine PDF call. This API not only lets you combine two PDFs, it also lets you specify a page range when combining, which means we can take our “prepend” PDF, take our larger PDF document, combine them, and specify a page range for the later to only get the specified number of sample pages.
Here’s what that code looks like:
In the body of the call, we pass in two assets, with the first representing the PDF “prepended” in front of our result and the second being a ‘slice’ of the main PDF.
This API call, like others in Acrobat Services, returns a job URL we can ping for our status update. The pollJob
function handles hitting this endpoint once a second and waiting until the job succeeds or fails. (Note that this example doesn’t actually handle errors, and usually it should!)
The final bit handles the download. Our job, when successful, returns the URL where the bits can be downloaded, so this is just passed to downloadFile
:
That was quite a bit of code to show you, so if you want to review, the entire application may be found here: https://github.com/cfjedimaster/document-services-demos/tree/main/article_support/book_demo
Here’s a screenshot from one of the samples, showing the first and second pages:
In this article, we demonstrated a process to automate a fairly common requirement for stores that sell digital documents — creating a sample PDF. However, there’s room for improvement.
The PDF prepended in front of the document is the same for all samples. What if we could personalize it and make it more specific to the source PDF being sampled? In the next article, we’ll show just that, making use of the powerful Document Generation API